Resources
Summary
Element Parameter Filter
Have you Ever wanted to Get your elements in Revit API based on a specific parameter value? And if you did you might have come across ElementParameterFilter Class.
So in this lesson I will break down how to use ElementParameterFilter so you can filter your elements based on parameter values.
And we are going to make 2 functions
Get Elements by Type Name.
Get Elements by Family Name
Let's start by exploring Documentation
Revit API Docs: Element Parameter Filter
Once we start exploring this class in documentation you will notice that we have a C# Example
And here is python translation of it:
Based on that example we can see that we need to prepare a rule to create our filter. And while there are different classes for rules depending on StorageType, in this example they use FilterDoubleRule
To create this rule we need
Evaluator (FilterNumericGreater)
Rule Value (What is the desired parameter value)
Tollerance (How far Element's values can be from yours)
There is also an option to invert the filter to get the opposite results. For that we just need to provide True boolean as a second argument when we create ElementParameterFilter
Let's start from the beginning and explore all required classes in more detail.
Filter Rules
When we look at ElementParameterFilter, we can see that it takes FilterRule as an argument. This is a base class for many other classes that we should use instead.
To choose correct one, we need to think of a StorageType of our parameter:
💡 Also if you want to check your Yes/No parameters, you should use FilterIntegerRule, since Yes/No are represented as 1/0 values.
These Elements have very similar constructors, but also there might be differences like CaseSensetive / Tollerance.
Here are Constructors (You Can Scroll Through Carousell):
Compare these arguments to Revit UI
Before we are going to break down each argument, let's compare them to Revit UI so you get more comfortable.
Have a look at Filter Rules that we create for Views.
Usually we select the following:
Category
Parameter
Evaluator (Contains, Equals…)
Rule Value
Do you see similarities to what we need for creating Revit API Rules? Except for Category, this is done separately in FEC class.
ParameterValueProvider
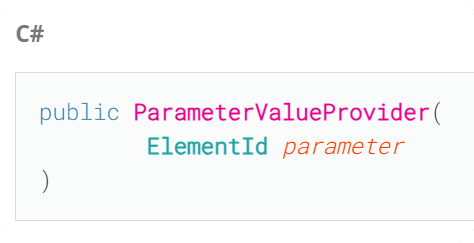
Evaluator
Next we need to select a class for evaluator. There are separate classes for Strings and Numeric, so make sure you choose the right one based on your parameter.
String
They all have no arguments, so we just select the one we want, and create an instance of it like this:
I choose Contains evaluator, so I can partially provide Rule Value to match.
Rule Value
Rule value can be anything that you want. In my case I want to create a function to get elements by Type Name, so I will write that in here!
FilterStringRule
Now we can combine all these arguments to create FilterStringRule
⚠️ But before we do that I want to warn you!
There was a change in Revit 2022 for this class, so we need to protect our script from breaking if we go below this version. And it's not complicated, as they just removed 4th argument for case-sensitivity.
It's also great to practice, how to make script for multiple Revit versions due to changes.
I will just get Revit version from app variable, and make 2 different code snippets for making this rule.
Now we have created FilterStringRule.
Create ElementParameterFilter
Lastly, we can use this rule to create ElementParameterFilter.
Let's also apply it to a collector to get ElementIds of Window Instances.
ElementParameterFilter for String Parameters
Now, let's summarize it all together into 1 snippet for Strings.
💡 Now you know all the steps, and it doesn't look that complicated.
It does take quite a few lines of code, but overall it's very simple and similar to Revit View filters, as it needs same things.
ElementParameterFilter for Numeric Parameters
Now let's also create a snippet for a numeric parameter.
1️⃣ We will take WALL_USER_HEIGHT_PARAM, to check wall heights.
2️⃣ I will choose FilterNumericGreater to only get walls that are higher
3️⃣ We will provide 10ft height. Be sure to convert if you use metric units!
4️⃣ Combine these parameters into a rule, and don't forget 4th argument for tollerance
5️⃣ Lastly, we can create ElementParameterFilter
6️⃣ And same as before, we can apply it to a collector
💡 Now it becomes so much easier, as we just need to tweak a few things. Just like in Revit UI when you duplicate a filter and change parameter and its value!
Turn it into a function:
🎦 Lastly, I want to give you a snippet from the video so you can see how it can easily be turned into a reusable function
Here is the snippet to get elements by Type Name:
💡 You can extend the functionality by adding second argument for evaluator to make it even more versatile!
HomeWork
Try to create your own ElementParameterFilter.
Choose a parameter, evaluator and a value to check, and see if you can get your elements using it.
Feel free to copy my snippet and modify it, as it will save you a lot of time!
⌨️ Happy Coding!